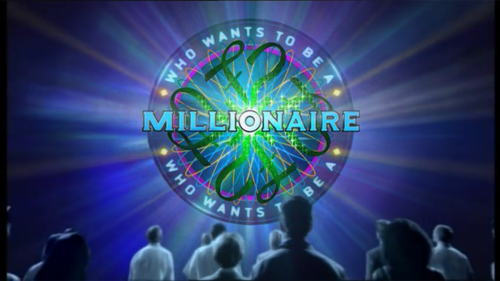
Creating a game based on the popular quiz show "Who Wants to Be a Millionaire" is an excellent way to practice JavaScript skills while building something fun and interactive. This blog post will walk you through how to build this game, complete with a timer, lifelines, and a scoring system.
Key Features of the Game
- Multiple Choice Questions: Players can select answers from four options.
- Timer: Players have 30 seconds to answer each question.
- Lifelines: Players can use lifelines such as 50:50, Ask the Audience, and Call a Friend.
- Scoring System: Players earn points based on the number of questions answered correctly.
- Audio Effects: The game features sound effects for correct and wrong answers, as well as background music.
Best Practices
- Code Organization: Keep your JavaScript code modular and well-structured.
- Use of Comments: Add comments to your code for better readability.
- Responsive Design: Ensure your game is playable on both desktop and mobile devices.
- User Input Validation: Validate user inputs to avoid errors during gameplay.
Step-by-Step Implementation
Step 1: Setting Up the Project
Create the following files in your project directory:
index.html
style.css
script.js
questions.json
(for the questions)- Audio files for background music and effects.
Step 2: HTML Structure
Here’s a simple HTML structure for the game:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta name="author" content="Your Name"> <title>Who Wants To Be A Millionaire</title> <link rel="stylesheet" href="style.css"> </head> <body> <main class="container"> <!-- Start Page --> <section class="start-box custom"> <div class="game-logo"> <img src="img/logo.png" alt="logo"> </div> <div class="instruction"> <h3>Instructions</h3> <ul class="instruction-list"> <li>30 seconds to answer each question</li> <li>Game over conditions include: <ul> <li>Time elapses</li> <li>Answer is wrong</li> <li>All questions have been answered</li> </ul> </li> <li>Guarantee prizes at questions 1, 10, 15</li> <li>Wrong answer results in winning the last guaranteed prize</li> <li>Lifelines available: <ul> <li>50:50 => Removes two wrong answers</li> <li>Ask the Audience</li> <li>Call a Friend</li> </ul> </li> </ul> </div> <div class="user-info"> <div class="start-game-btn btn">Start game</div> </div> </section> <!-- Main Game Page --> <section class="game-box custom"> <div class="game"> <div class="timer">30</div> <div class="current-question-amount"></div> <div class="life-line-display-box"> <div class="call"> <img src="img/call.png" alt="call"> <div class="call-answer"></div> </div> <div class="au-cover"> <h4 class="alpha">ABCD</h4> <div class="au-container"> <div class="au-box" id="0"><span></span><div class="bx"></div></div> <div class="au-box" id="1"><span></span><div class="bx"></div></div> <div class="au-box" id="2"><span></span><div class="bx"></div></div> <div class="au-box" id="3"><span></span><div class="bx"></div></div> </div> </div> </div> <div class="question"> <div class="question-text"></div> <div class="options"> <div class="option" id="0">Option 1</div> <div class="option" id="1">Option 2</div> <div class="option" id="2">Option 3</div> <div class="option" id="3">Option 4</div> </div> </div> <div class="next-question-btn btn">Next Question</div> <div class="playAgain-btn btn">Play Again</div> <div class="amount-won"></div> </div> </section> </main> <script src="script.js"></script> </body> </html>
Step 3: CSS Styling
Here’s a basic CSS structure to style your game:
css body { font-family: Arial, sans-serif; background-color: #f4f4f4; margin: 0; padding: 0; } .container { width: 100%; max-width: 800px; margin: auto; } .start-box, .game-box { display: none; padding: 20px; background-color: white; border-radius: 5px; box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1); } .btn { background-color: #5cb85c; color: white; padding: 10px 15px; border: none; border-radius: 5px; cursor: pointer; } .btn:hover { background-color: #4cae4c; }
Step 4: JavaScript Logic
Below is a simplified version of the JavaScript code to manage the game’s logic:
javascript let MainThemePlay = new Audio("audio/main_theme.mp3"); let wrongPlay = new Audio("audio/wrong.mp3"); let correctPlay = new Audio("audio/correct.mp3"); let callPlay = new Audio("audio/call.mp3"); let fifty50Play = new Audio("audio/5050.mp3"); let audiencePlay = new Audio("audio/audience.mp3"); let inGamePlay = new Audio("audio/64000_music.mp3"); // Selectors let startBox = document.querySelector(".start-box"); let startBtn = document.querySelector(".start-game-btn"); let gameBox = document.querySelector(".game-box"); let timer = document.querySelector(".timer"); let questionText = document.querySelector(".question-text"); let options = document.querySelectorAll(".option"); let nextQuestionBtn = document.querySelector(".next-question-btn"); let amountWon = document.querySelector(".amount-won"); let questionIndex = 0; let quiz = []; // Fetch questions from questions.json fetch("questions.json") .then(response => response.json()) .then(data => { quiz = data; startGame(); }) .catch(error => console.error(error)); // Start the game function startGame() { startBox.style.display = "none"; gameBox.style.display = "block"; generateQuestion(); startTimer(); } // Generate a question function generateQuestion() { if (questionIndex < quiz.length) { questionText.textContent = quiz[questionIndex].question; options.forEach((option, index) => { option.textContent = quiz[questionIndex].options[index]; option.onclick = checkAnswer; }); } else { // End the game and show the result endGame(); } } // Check the selected answer function checkAnswer(event) { const selectedOption = event.target; if (selectedOption.textContent === quiz[questionIndex].answer) { correctPlay.play(); questionIndex++; generateQuestion(); } else { wrongPlay.play(); endGame(); } } // Start timer function startTimer() { let timeLeft = 30; timer.textContent = timeLeft; const countdown = setInterval(() => { timeLeft--; timer.textContent = timeLeft; if (timeLeft <= 0) { clearInterval(countdown); wrongPlay.play(); endGame(); } }, 1000); } // End the game function endGame() { gameBox.style.display = "none"; amountWon.textContent = "Game Over!"; amountWon.style.display = "block"; } // Start button event listener startBtn.addEventListener("click", () => { startGame(); });
Step 5: Questions JSON File
You need to create a questions.json
file containing your quiz questions in the following format:
json [ { "question": "What is the capital of France?", "options": ["Berlin", "Madrid", "Paris", "Lisbon"], "answer": "Paris" }, { "question": "Which planet is known as the Red Planet?", "options": ["Earth", "Mars", "Jupiter", "Saturn"], "answer": "Mars" } // Add more questions as needed ]
Conclusion
By following these steps, you can create a fully functional "Who Wants to Be a Millionaire" game using JavaScript. Feel free to customize the game further by adding more features or improving the user interface.
Source Code
Download Source Code