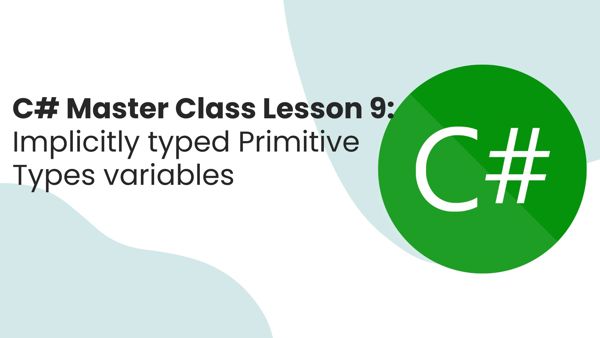
In C#, variables can either be implicitly typed or explicitly typed. Understanding the differences between these, especially with respect to primitive types, is important for writing clear and efficient code.
1. Implicitly Typed Variables
An implicitly typed variable uses the var
keyword. When you declare a variable using var
, the compiler determines its type based on the assigned value. However, once the type is inferred, it cannot be changed later.
Syntax:
csharp var variableName = value;
The compiler infers the data type based on the value on the right-hand side of the assignment.
Example 1: Implicitly Typed with Integer
csharp var number = 10; // Compiler infers that 'number' is of type int Console.WriteLine(number.GetType()); // Output: System.Int32
Here, the compiler detects that number
is an int
(which is a primitive type), because 10
is an integer.
Example 2: Implicitly Typed with String
csharp var name = "Alice"; // Compiler infers that 'name' is of type string Console.WriteLine(name.GetType()); // Output: System.String
Since the value "Alice"
is a string, the compiler infers that the type of name
is string
.
Example 3: Implicitly Typed with a Complex Type
csharp var customer = new Customer(); // Compiler infers 'customer' is of type Customer
Here, var
is used with a more complex type (Customer
), and the type is inferred from the new Customer()
object creation.
Limitations of Implicit Typing:
- Initialization Required: You must initialize an implicitly typed variable at the point of declaration because the compiler needs the value to infer the type.
csharp var x; // Error: Cannot use 'var' without an initializer
- Readability: Using
var
excessively can make code harder to read, especially when the inferred type isn’t obvious from the value assigned.
2. Primitive Types
Primitive types in C# are the most basic data types. These include types like int
, float
, double
, bool
, char
, etc. Primitive types are value types, which means they store their data directly in memory, and they are predefined by the C# language.
Common Primitive Types in C#:
int
: Represents a 32-bit signed integer.double
: Represents a 64-bit floating-point number.bool
: Represents a Boolean value (true
orfalse
).char
: Represents a single 16-bit Unicode character.
Explicitly Typed Primitive Variables:
Explicitly typing variables means declaring the type explicitly.
Example 1: Explicitly Typed Integer
csharp int age = 25; Console.WriteLine(age); // Output: 25
In this case, you explicitly declare age
as an int
. This makes the code immediately clear to anyone reading it.
Example 2: Explicitly Typed Boolean
csharp bool isCompleted = true; Console.WriteLine(isCompleted); // Output: True
Here, the isCompleted
variable is explicitly typed as bool
.
Example 3: Explicitly Typed Character
csharp char initial = 'A'; Console.WriteLine(initial); // Output: A
This declares the initial
variable as a char
, which can store a single Unicode character.
Combining Implicitly Typed with Primitive Types
While var
is commonly used to simplify code, it can be used with primitive types as well. However, it doesn’t change how the primitive types behave. The type is still inferred by the compiler as the corresponding primitive type.
Example: Implicitly Typed Primitive Type
csharp var score = 98; // The compiler infers score is of type int Console.WriteLine(score.GetType()); // Output: System.Int32
In this example, the var
keyword is used, but the type inferred by the compiler is int
, which is a primitive type.
Why Use Implicit Typing?
- Code Clarity in Complex Types: When the type is obvious from the context or the right-hand side, implicit typing with
var
can make the code cleaner.
csharp var products = new List<Product>();
- Reduces Repetition: For long type names, using
var
reduces repetition and makes the code more concise.
csharp Dictionary<string, List<int>> myDictionary = new Dictionary<string, List<int>>(); // vs var myDictionary = new Dictionary<string, List<int>>();
When to Avoid Implicit Typing?
- Primitive Types: For primitive types, it's often better to be explicit to make the code more readable. Using
var
forint
,bool
, orchar
can make the code less readable.
csharp Copy code int counter = 0; // Clear and easy to understand var counter = 0; // Less clear at a glance
- Unclear Types: When the type being assigned isn't immediately obvious, it's better to use explicit typing for clarity.
csharp Copy code var result = DoSomething(); // What type is result?
Conclusion
- Implicitly typed variables (
var
) allow the compiler to determine the type based on the assigned value, reducing verbosity. - Primitive types are basic data types like
int
,bool
,char
, etc., and they are explicitly declared unless inferred byvar
. - While implicit typing can simplify complex type declarations, for primitive types, it’s often clearer to declare them explicitly.