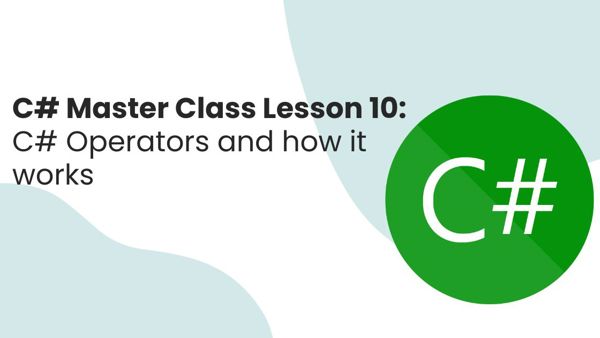
In C#, operators are special symbols or keywords that perform operations on operands (variables or values) to produce a result. Understanding how operators work is fundamental to programming in C#. Let's break down different types of operators with real-life examples to understand how they function in practical scenarios.
Types of Operators in C#
C# supports various types of operators, which can be categorized as follows:
- 1. Arithmetic Operators
- 2. Relational (Comparison) Operators
- 3. Logical Operators
- 4. Assignment Operators
- 5. Unary Operators
- 6. Bitwise Operators
- 7. Ternary Operator
1. Arithmetic Operators
Arithmetic operators perform mathematical operations. They include:
+
(Addition)-
(Subtraction)*
(Multiplication)/
(Division)%
(Modulus)
Real-Life Example: Calculating Total Shopping Cost
Let’s say you are building an application to calculate the total cost of items in a shopping cart.
csharp int itemPrice = 500; // Price of one item int quantity = 4; // Number of items bought int totalCost = itemPrice * quantity; // Total cost of shopping Console.WriteLine(totalCost); // Output: 2000
Here, the *
operator multiplies the price of a single item by the quantity purchased to calculate the total cost.
Example: Calculating Modulus
You can also use the modulus operator %
to determine the remainder of a division.
csharp int totalBill = 200; int numberOfPeople = 3; int remainder = totalBill % numberOfPeople; // Remainder when dividing bill among people Console.WriteLine(remainder); // Output: 2
This tells you how much will be left over after equally dividing the total bill by the number of people.
2. Relational (Comparison) Operators
Relational operators compare two values or variables and return a Boolean result (true
or false
). These include:
==
(Equal to)!=
(Not equal to)>
(Greater than)<
(Less than)>=
(Greater than or equal to)<=
(Less than or equal to)
Real-Life Example: Age Eligibility for Driving License
You can use relational operators to check if a person is eligible to apply for a driving license.
csharp int age = 18; bool isEligible = age >= 18; Console.WriteLine(isEligible); // Output: True
Here, the >=
operator checks whether the person’s age is greater than or equal to 18, which is the minimum driving age in many countries.
3. Logical Operators
Logical operators are used to combine multiple conditions. They include:
&&
(Logical AND)||
(Logical OR)!
(Logical NOT)
Real-Life Example: Loan Application Approval
Let’s say you are building an application to approve loan applications based on conditions like income and credit score.
csharp int income = 50000; int creditScore = 700; bool isEligibleForLoan = income > 30000 && creditScore > 650; Console.WriteLine(isEligibleForLoan); // Output: True
Here, the &&
operator checks if both conditions (income greater than 30,000 and credit score greater than 650) are true for the loan to be approved.
4. Assignment Operators
Assignment operators are used to assign values to variables. The basic assignment operator is =
. There are also compound assignment operators:
+=
(Add and assign)-=
(Subtract and assign)*=
(Multiply and assign)/=
(Divide and assign)%=
(Modulus and assign)
Real-Life Example: Incrementing a Salary
Imagine you are building an HR system where employees get annual salary increments.
csharp int salary = 50000; salary += 5000; // Increment salary by 5000 Console.WriteLine(salary); // Output: 55000
In this example, the +=
operator increments the salary by 5,000, combining addition and assignment in a single operation.
5. Unary Operators
Unary operators operate on a single operand. Common unary operators include:
++
(Increment)--
(Decrement)+
(Unary plus)-
(Unary minus)!
(Logical NOT)
Real-Life Example: Stock Quantity Management
You might need to increase or decrease the stock of an item in an inventory system.
csharp int stock = 10; stock++; // Increment stock by 1 Console.WriteLine(stock); // Output: 11 stock--; // Decrement stock by 1 Console.WriteLine(stock); // Output: 10
The ++
operator increments the stock quantity by 1, while the --
operator decreases it by 1.
6. Bitwise Operators
Bitwise operators operate at the binary level. They include:
&
(AND)|
(OR)^
(XOR)~
(NOT)<<
(Left shift)>>
(Right shift)
Real-Life Example: Access Control System
Bitwise operators can be useful in systems where different permissions are encoded as bits. For instance, in an access control system:
csharp int readPermission = 1; // 0001 int writePermission = 2; // 0010 int executePermission = 4; // 0100 int currentPermissions = readPermission | writePermission; // Combine read and write permissions using OR Console.WriteLine(currentPermissions); // Output: 3 (0011) bool hasWritePermission = (currentPermissions & writePermission) != 0; // Check if write permission is granted using AND Console.WriteLine(hasWritePermission); // Output: True
This example combines read and write permissions using the bitwise OR operator (|
) and checks if the user has write permission using the bitwise AND operator (&
).
7. Ternary (Conditional) Operator
The ternary operator (?:
) is a compact form of an if-else
statement. It evaluates a condition and returns one of two values depending on whether the condition is true
or false
.
Real-Life Example: Grading System
Let’s build a simple grading system that assigns "Pass" or "Fail" based on the student’s score.
csharp int score = 75; string result = score >= 50 ? "Pass" : "Fail"; Console.WriteLine(result); // Output: Pass
In this case, the ternary operator checks if the score
is greater than or equal to 50. If true, it returns "Pass"; otherwise, it returns "Fail."
Conclusion
C# operators are powerful tools that enable you to perform a wide variety of tasks, from basic arithmetic to complex logical decisions. Here’s a quick recap:
- Arithmetic operators are used for mathematical calculations.
- Relational operators compare values.
- Logical operators combine multiple conditions.
- Assignment operators assign values and modify them.
- Unary operators perform operations like incrementing and negating.
- Bitwise operators manipulate binary data.
- The ternary operator is a concise way to implement conditional logic.
By mastering these operators, you can efficiently build real-world applications in C#.