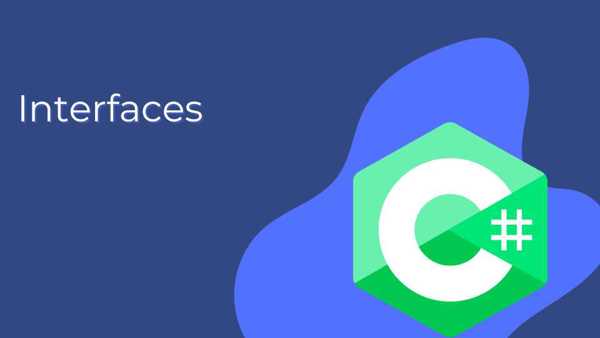
1. What is Interface Inheritance?
Interface inheritance in C# allows one interface to inherit from another interface. This means the derived interface will include all the members (methods, properties, events) of the base interface, and it can also add new members. It’s like a child inheriting traits from a parent but also having its own unique traits.
2. Layman’s Explanation with Real-Life Example
Think of a vehicle hierarchy:
- A
Vehicle
interface defines basic functionality likeStart()
andStop()
. - A
Car
interface inherits fromVehicle
and adds its own functionality likeAccelerate()
. - A
SportsCar
interface inherits fromCar
and adds even more specific functionality likeTurboBoost()
.
This way, you can build specialized interfaces without duplicating code.
3. Complete Solution: Real-World Project Example
Let’s build a vehicle management system using interface inheritance. We’ll define a base interface IVehicle
and derive more specialized interfaces like ICar
and ISportsCar
.
Step 1: Define the Base Interface
The IVehicle
interface defines basic functionality for all vehicles.
public interface IVehicle { void Start(); void Stop(); string GetFuelType(); }
Step 2: Define Derived Interfaces
The ICar
interface inherits from IVehicle
and adds car-specific functionality.
public interface ICar : IVehicle { void Accelerate(); void Brake(); }
The ISportsCar
interface inherits from ICar
and adds sports car-specific functionality.
public interface ISportsCar : ICar { void TurboBoost(); }
Step 3: Implement the Interfaces
Now, let’s create a class SportsCar
that implements the ISportsCar
interface.
public class SportsCar : ISportsCar { public void Start() { Console.WriteLine("Sports car started."); } public void Stop() { Console.WriteLine("Sports car stopped."); } public string GetFuelType() { return "Premium Unleaded"; } public void Accelerate() { Console.WriteLine("Sports car accelerating."); } public void Brake() { Console.WriteLine("Sports car braking."); } public void TurboBoost() { Console.WriteLine("Sports car turbo boost activated!"); } }
Step 4: Use the Interfaces in a Program
Let’s create a program to demonstrate how interface inheritance works.
class Program { static void Main(string[] args) { // Create an instance of SportsCar SportsCar mySportsCar = new SportsCar(); // Use IVehicle methods mySportsCar.Start(); mySportsCar.Stop(); Console.WriteLine($"Fuel type: {mySportsCar.GetFuelType()}"); // Use ICar methods mySportsCar.Accelerate(); mySportsCar.Brake(); // Use ISportsCar methods mySportsCar.TurboBoost(); } }
Step 5: Output
When you run the program, you’ll see the following output:
Copy
Sports car started. Sports car stopped. Fuel type: Premium Unleaded Sports car accelerating. Sports car braking. Sports car turbo boost activated!
Step 6: Explanation
- Interface Inheritance:
ICar
inherits fromIVehicle
, so it includesStart()
,Stop()
, andGetFuelType()
.ISportsCar
inherits fromICar
, so it includes all methods fromICar
andIVehicle
, plus its ownTurboBoost()
method.- Implementation:
- The
SportsCar
class implementsISportsCar
, so it must provide implementations for all methods inIVehicle
,ICar
, andISportsCar
.
Step 7: Best Practices and Features
- Best Practices:
- Use interface inheritance to avoid code duplication and create a clear hierarchy.
- Keep interfaces small and focused (Single Responsibility Principle).
- Use meaningful names for interfaces and methods.
- Avoid deep inheritance hierarchies (prefer composition over inheritance).
- Document the purpose of each interface clearly.
- Features:
- Allows you to build specialized interfaces without rewriting code.
- Promotes loose coupling and modular design.
- Enables polymorphism (e.g., treat
SportsCar
asIVehicle
,ICar
, orISportsCar
).
Step 8: Pros and Cons
- Pros:
- Code reusability: Derived interfaces inherit members from base interfaces.
- Flexibility: You can create specialized interfaces without modifying existing ones.
- Polymorphism: Objects can be treated as their base or derived interface types.
- Cons:
- Can lead to complex hierarchies if overused.
- Deep inheritance chains can make the code harder to understand.
Step 9: Alternatives
- Abstract Classes: Use abstract classes if you need to provide default implementations for some methods.
- Composition: Instead of inheritance, use composition to combine functionality from multiple interfaces or classes.
Step 10: When to Use Interface Inheritance
- Use interface inheritance when:
- You need to create a hierarchy of related interfaces.
- You want to enforce a contract across multiple levels of specialization.
- You want to avoid duplicating code in related interfaces.
Step 11: Complete Solution Code
Here’s the complete code for the solution:
using System; // Base interface public interface IVehicle { void Start(); void Stop(); string GetFuelType(); } // Derived interface public interface ICar : IVehicle { void Accelerate(); void Brake(); } // Further derived interface public interface ISportsCar : ICar { void TurboBoost(); } // Implementation public class SportsCar : ISportsCar { public void Start() { Console.WriteLine("Sports car started."); } public void Stop() { Console.WriteLine("Sports car stopped."); } public string GetFuelType() { return "Premium Unleaded"; } public void Accelerate() { Console.WriteLine("Sports car accelerating."); } public void Brake() { Console.WriteLine("Sports car braking."); } public void TurboBoost() { Console.WriteLine("Sports car turbo boost activated!"); } } // Program class Program { static void Main(string[] args) { // Create an instance of SportsCar SportsCar mySportsCar = new SportsCar(); // Use IVehicle methods mySportsCar.Start(); mySportsCar.Stop(); Console.WriteLine($"Fuel type: {mySportsCar.GetFuelType()}"); // Use ICar methods mySportsCar.Accelerate(); mySportsCar.Brake(); // Use ISportsCar methods mySportsCar.TurboBoost(); } }