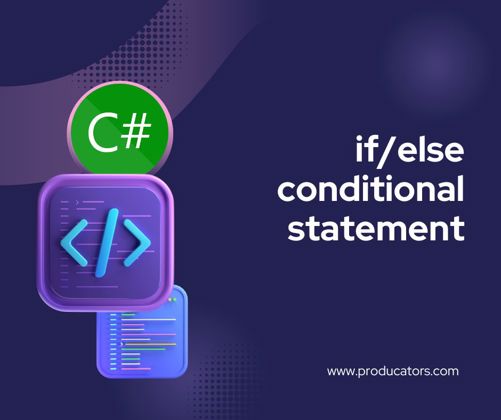
Understanding the if/else
Conditional Statement in C#
In programming, conditional statements are critical for controlling the flow of a program based on certain conditions. One of the most commonly used conditional statements in C# is the if/else
statement, which allows developers to execute specific blocks of code depending on whether a given condition evaluates to true
or false
.
In real-life scenarios, conditional logic is used constantly to make decisions. For instance, "If it's raining, take an umbrella. Else, go without one." This is exactly what the if/else
statement in C# does—it decides which code to run based on whether a condition is met.
Syntax of if/else
in C#
Here’s the basic structure of an if/else
statement in C#:
csharp if (condition) { // Code to be executed if the condition is true } else { // Code to be executed if the condition is false }
- Condition: An expression that evaluates to either
true
orfalse
. - If block: Executed if the condition is
true
. - Else block: Executed if the condition is
false
.
Example 1: Simple if/else
Statement
csharp int temperature = 30; if (temperature > 25) { Console.WriteLine("It's a hot day, drink plenty of water!"); } else { Console.WriteLine("The weather is cool."); }
Breakdown:
- If the
temperature
is greater than 25, the message "It's a hot day, drink plenty of water!" will be printed. - Otherwise, the message "The weather is cool." will be printed.
Real-Life Example
Imagine you're at a traffic light:
- If the light is green, you proceed.
- If the light is red, you stop.
Here’s how this logic looks in C#:
csharp string trafficLight = "green"; if (trafficLight == "green") { Console.WriteLine("You can proceed."); } else { Console.WriteLine("Stop! Wait for the light to turn green."); }
else if
Statement
Sometimes, you need to evaluate multiple conditions. This is where the else if
statement comes in handy. You can chain multiple conditions to make more complex decisions.
Syntax of else if
:
csharp if (condition1) { // Code if condition1 is true } else if (condition2) { // Code if condition2 is true } else { // Code if both conditions are false }
Example 2: Using else if
for Multiple Conditions
csharp int age = 20; if (age < 13) { Console.WriteLine("You are a child."); } else if (age >= 13 && age <= 19) { Console.WriteLine("You are a teenager."); } else { Console.WriteLine("You are an adult."); }
Here, the code checks multiple conditions to determine whether a person is a child, teenager, or adult.
Real-Life Scenario for else if
Consider a store offering discounts:
- If you spend more than $100, you get a 20% discount.
- If you spend more than $50, you get a 10% discount.
- Otherwise, you get no discount.
This can be expressed in code:
csharp int totalAmount = 75; if (totalAmount > 100) { Console.WriteLine("You get a 20% discount!"); } else if (totalAmount > 50) { Console.WriteLine("You get a 10% discount."); } else { Console.WriteLine("No discount available."); }
Best Practices for if/else
Statements
- Keep Conditions Simple: Avoid complex conditions. If a condition is too complicated, consider refactoring it into smaller functions.
- Example: Break down long conditional expressions into meaningful boolean variables.
- Use Ternary Operator for Simple Decisions: For simple
if/else
logic, you can use the ternary operator to make your code more concise.
csharp string result = (score >= 50) ? "Pass" : "Fail";
- Avoid Deep Nesting: Multiple nested
if/else
statements can make your code difficult to read. Consider using early exits (return
,break
, etc.) where applicable to reduce nesting. - Short-Circuit Evaluation: In conditions with multiple expressions, C# will stop evaluating as soon as it knows the result. Use this to your advantage for performance optimizations.
csharp if (x != 0 && y / x > 1) { // Safe because x != 0 is evaluated first }
- Use
else
Only When Necessary: If the condition can be inferred from the firstif
statement, you may not need anelse
block.
Features of if/else
Statements
- Conditionally Executes Code: Allows your program to take different actions based on conditions.
- Supports Multiple Conditions: You can chain multiple
else if
blocks to check multiple conditions. - Handles Logical Operators: Conditions can use logical operators such as
&&
(AND),||
(OR), and!
(NOT). - Ternary Operator Support: For compact
if/else
logic, C# provides the ternary operator? :
. - Short-Circuit Evaluation: When evaluating complex conditions, C# stops checking conditions as soon as the result is determined.
Build a Simple Application
Let's build a simple console-based grading system using if/else
statements. The system will prompt the user to enter their score and will return a grade based on the score.
csharp using System; public class GradingSystem { public static void Main(string[] args) { Console.WriteLine("Enter your score: "); int score = Convert.ToInt32(Console.ReadLine()); if (score >= 90) { Console.WriteLine("Your grade is A."); } else if (score >= 80) { Console.WriteLine("Your grade is B."); } else if (score >= 70) { Console.WriteLine("Your grade is C."); } else if (score >= 60) { Console.WriteLine("Your grade is D."); } else { Console.WriteLine("Your grade is F."); } } }
Explanation:
- The user is prompted to enter their score.
- Depending on the score, the program will return a grade ranging from A to F.